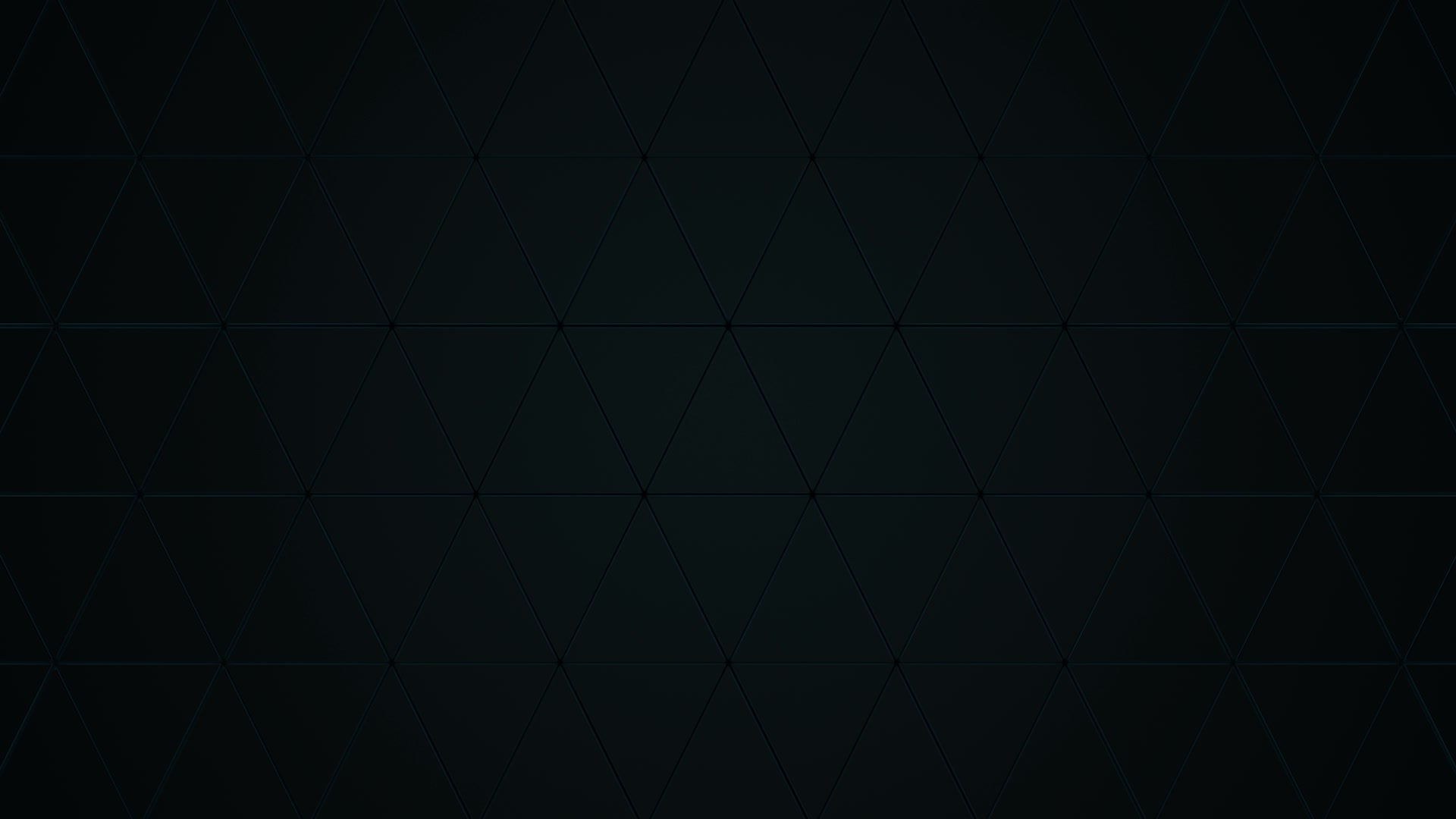

TurnBased Timeline System
Inspired by many famous turn-based games such as the Fire Emblem series, XCOM series as well as the the 2D RPG game Child Of Light, I decided to learn, design and make a modular 3D turn based system that can be used for multiple purposes.
This system contains three main features that can be found in the games I mentioned above:
-
The Grid Movement
-
The Action System
-
The Turn System
-
The Timeline System.
1 - The Grid System
This grid system is designed and build based on two tutorials from Cody Monkey
There are three major components for this grid system: the grid object, the grid position and the grid system, relations between these three script types is shown in the figure01 below
Figure 01: Three components of the grid movement system and their relations
1.1-Grid Object Class
The grid object refers to every individual grid in the map, as shown in the figure 02 below
Each grid object will record:
-
Which grid system it belongs to
-
The grid position
-
A list that hold all the units that currently belongs to this grid
The functions of the grid object are:
-
Add or remove unit from its list
-
Return the unit stand on it
-
Return bool whether this grid is occupied or not
Figure 02: Grid Object
1.2-Grid Position Class
The grid position class is used to store the vertical(z) and horizontal(x) position of the grid object, as shown in the figure 03 below
The grid position class will record:
-
The x position of the grid object
-
The z position of the grid object
The functions of the grid position are:
-
Return if two grid positions are equals to eachother
-
Return the grid position in string format
Figure 03: Grid Position Class
1.3-Grid System Class
The Grid System class is used generate the actual grid system into the game, it hold the x and z length of the map as well as the size of each grid. It also contians a 2D array that populate and record the GridObject and the GridPosition for each single grid in the map. As shown in the figure 04 below
The Grid System Class will define:
-
The length of the map on x axis
-
The length of the map on z axis
-
The size of each individual grid
-
A 2d array that stores all the grid objects inside this gridsystem
The functions of the Grid System Class are:
-
Generate the grid system for the game
-
Spawn grid objects on each grid position
-
Return the grid object on certain grid position
-
Return the world position of the grid object
-
Check if a given grid position is inside the grid system
-
Return the width and length of the grid system
When creating the grid object on each grid position, the system will loop through all the x and z positions and
-
First, generate grid position (x,z) for this grid and record
-
Second, create the gridobject based on the grid position and sign this grid system to the grid object
-
Last step, add the gridobject with its grid position into the 2d array list:
-
gridobjectArray[x,z] = createGridObject(gridSystem, gridPosition);
-
Figure 04: Grid System Class - Constructor
2 - The Action System
The design intention is to create a modular action system that allow the developers to easily create different actions to fit different needs and ideas in game. There are two core class for this system: The Base Action Class and The UnitActionSystem Class
2.1-The Base Action Class
Base action class holds all the basic logic and universal functions for all actions. All actions created in game will be inherit from this class.
The Base Action Class will define: (Figure 05)
-
A protected bool used to check if this action is active or not
-
A protected action used to called when the action is completed
-
A unitBasic variable that record which unit this action belongs to
-
Two event that will called when
-
OnAnyActionStarted
-
OnAnyActionCompleted
-
The functions of the Base Action Class are: (Figure 06)
-
An abstract function used to let unit take action
-
A virtual function that returns the action cost
-
A protected action called when action started
-
A protected action called when action completed
-
A function that returns a list with all the valid grid positions for this action
Figure 05 / 06: Base Action Class
2.2-Action Class Example:
Attack Action
Attack Action is used to let one unit attack against another unit in game. Three questions need to be answer in order to make it funciton properly:
-
Who to attack?
-
When to attack?
-
How to attack?
2.2.1-Who to Attack?
Unit will try to detect the potential attackable unit within its own attack range by overwriting the GetValidGridPositionList Funciton from the Base Action Class
Function will loop through all the grid positions around the unit & within its attack range and check for the following conditions:
-
If that grid is within the map, if so, next step
-
If that grid has no other friendly unit stand on it, if not, next step
-
If there is any enemy stand on it, if so, next step
-
If there is no obstacles between the unit and the enemy, if not, next step
-
If all the tests passed, add this grid as attakable position
Figure 07: Getting Valid Attack Targets
2.2.2-When to Attack?
Once there is any enemies found based on the test from the previous step, player is able to get the unit from that grid posiiton and call TakeAction function is start attack
Figure 08: TakeAction Function
2.2.3-How to Attack?
State Machine is used to control each step of the attack action. As shown in the previous step, when the TakeAction function is called, The turning state will be called.
There are three state for the attack action in total:
The Turning State: Turn the current unit toward the attack target
The Slashing State: Play Attack animation and apply damage to the player
Cooloff State: End aciton and call the OnActionComplete function
Once all the actions finished within each State, it will automatically switched to the next state
Figure 09: State Machine for Attack Action
2.3-UnitActionSystem
A singleton class that used for unit seletion and unit deselection as well as the unit action execution check. This purpose of creating this class is to have a singletion public class than can be accessed by all other scripts in game so that each actual action classes are better protected with other external access.
The UnitAction Class will record:
-
The current selected unit
-
The current selected action
-
Is the system executing action
-
Three events that will called when
-
The selected unit changed
-
The selected action change
-
Any Action start to execute
-
The functions of the Base Action Class are: (Figure 10 / 11)
-
Let the selected unit execute action
-
Select / deselect unit
-
Select /deselect action
-
Handle MouseClick
This script is the one that actually handle the user input and work as a bridge to called different funciton within each action or unit script accordingly.
Figure 10/11: Unit Selection and Action Selection inside UnitActionSystem Script
3-Turn System
In this system, the turn for player units are seperate from the enemy units.
For player units, once all the act points are used, the unit will not recharge its action points by itself. Player need to click the end turn button to end the turn for the current selected unit, and this unit will enter the energy recharge state until all action points are stored. As shown in the figure below.
Figure 12: Unit End its turn and Recharging
An UnitTurnTimer class has been created and attached to all the player controlled units.
This class defines the following variables:
-
Bool to check if the current turn is active or not
-
Float used for energy recharge timer
-
A reference to the player Tag object (Timeline)
Once the end turn button has been clicked, the timer will start and player can no longer control this unit anymore. As shown in the figure 13 below.
Figure 13: Turn System - Unit
For enemy units, once their action points are all consumed, the timer will start and recharge for next turn automatically, as shown in the figure below.
I use state machine to control the enemy behaviro, there are three states in total:
-
Waiting Phase
-
Taking action phase
-
Busy Phase
The Timer Count Down will happened in the waiting phase
Figure 14: Timer
4-TimeLine System
The timeline system is inspried by the game CHILD OF LIGHT and I think the idea of bring a timeline into the turn-based game is so good that it bring the strategitic play of the game into another level.
Figure 15: Timeline from CHILD OF LIGHT
4.1-UI Setup
An UI Image is used as the base of the progression bar, as shown in the first figure below, the yellow part represent the loading progress and the red part represent the cast part.
Unit tag prefabs are created to represent each unit on the progression bar. Once the unit is spawned into the game, the according unit tag will be also created and show up on the progression bar.
Figure 16: The UI setup for Timeline System
On game start, the system will go thorugh all the player units and the enemy units in the game and spawn their tag accordingly. The spawn location of the friend units and the enemies are seperated so that player is easy to tell, as shown in the figure below
Figure 17: Unit Tags Spawned
In terms of actually control the movement of each unit tag, a reference of the unit tag was make inside the UnitTurnTimer Script. The position of the tag will lerping between the start point and the end point of the progress bar, as shwon in the figure 18 and 19 below.
Figure 18: Recording the start and end position of the unit tags
Figure 19: Function used to update the location of the unit tags
Final Product
Epilogue
By working on the project, I had a lot practice on how to design modular systems to avoid massive repetition amoung scripts. An clean and highly modular system allows develper to easily design and explore new ideas and mechanics. Public Events, Actions as well as Generics are great tools I learned and used in this project which do gives me a chance to practice and imporve my coding skills in C#